As newbies to the software engineering field, most of us focus a lot on building web apps that are ‘working’ or at least ‘demo-able’, which is somewhat understandable. But I have seen most newbies into software engineering having various problems when they are supposed to complete a project within a certain time period. If you are a software engineering undergraduate, I guess you have experienced some issues related to your code, such as,
- Your code is giving a lot of compile errors.
- Your IDE (integrated development environment — simply the tool you use to code) shows so many red markings and alerts, and you can’t run your code.
- You can’t remember the execution flow of your code.
- Your project proposal contains some sets of diagrams that are opposite to your current implementation (and you have no idea how to modify your diagrams to reflect the current code you have).
- You can’t write unit tests for your code (Your methods/functions are not testable).
- When you look at your code, You don’t ‘feel good to read your code.
If so, you may continue reading this article to be inspired by “clean coding practices” meanwhile you are working on writing web applications to build great solutions in less time. And, of course, you will find the above problems are getting resolved without much additional effort!
If you are someone who wants to know What is “Clean Coding” and “How You Can Start Writing Clean Code”, this 5-minute article is for you.
What you are going to learn…
- How to Name Files in JavaScript
- How to Name Boolean Type Variables
- How to Name Private Variables or Functions/Methods
- How to Name Constants
- How to Name Functions
- How to Name Classes
- How to Name Components
- Some General Tips
In this article, I’ll guide you to write Clean Code from the basics. However, Clean Coding is a skill you can improve by practising it whenever possible. I’m using general examples using JavaScript, but the concepts are mostly similar in other programming languages/frameworks except for a few scenarios. Please follow official guidelines/documentation of your language for further details.
Let’s get started with one famous quote from Martin Fowler, an american software engineer and one of the founders of Agile Manifesto.
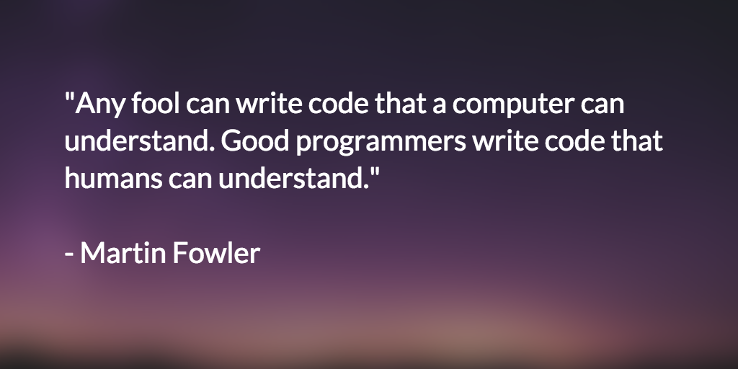
As a beginner, How can you improve your Clean Coding skills?
Let’s start with the basics of Naming JavaScript Variables and JavaScript Functions
Variables names are used to identify a value stored in memory locations instead of using memory addresses. So when you are supposed to define a variable, make sure to put a meaningful name instead of using a very generic or random name. Some examples of ‘not so good’ variable names are,
int i= 10,
String s = “abc”,
Student stu = new Student();
"There are only two hard things in Computer Science: cache invalidation and naming things." - Phil Karlton
— Programming Wisdom (@CodeWisdom) June 28, 2018
But I know, it’s easier said than done.
How do you verify whether your identifier it is ‘Good’?
Simple, If your name tells,
- Why It Exists
- What It Does
- How is it used
You have chosen a ‘Good’ name.
but If your name requires a comment to explain it, probably your name is ‘Not So Good’.
Let’s look into Clean Coding Practices related to JavaScript.
The below clean code practices apply to Angular, React, Vue or any other JS-based front-end frameworks as well.
Some good JS variable names,
var remainingTimeInDays;
var daysUntilShipment;
var daysSinceModification;
Did you know that in JavaScript, variable names are Case Sensitive?
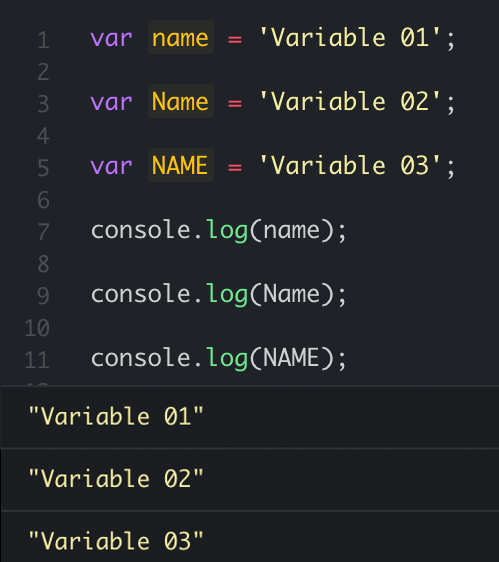
1. How to Name Files in JavaScript?
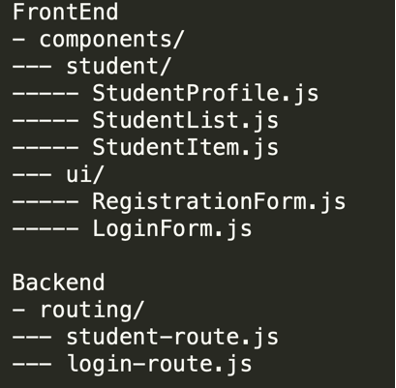
If you are going to name project files in any JavaScript framework, you may use PascalCase for frontend projects with JavaScript Frameworks like React. You can use kebab-case if you are working with a Backend Framework (Express/Node etc.).
- Important Note: This is a generic pattern you can follow in JavaScript-based frameworks. But file naming standards are different in other Non-JS based Frameworks. I advise you to refer to official style guides specific to your framework or use Google Style Guide to explore more. I have linked them at the end of this article.
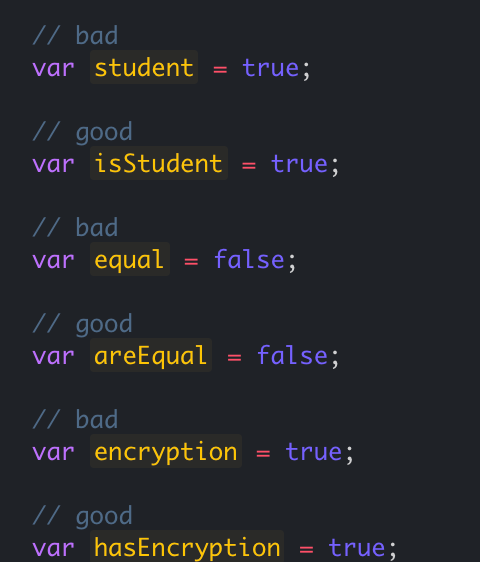
2. How to Name Boolean Type Variables?
When you are going to name a variable that defines a boolean type value, It is always good to give a name that can express the variable, whether it can be ‘true’ or ‘false’. One approach is to start with ‘is’ as a prefix. Ex: isStudent, isValid etc.
You may use other prefix terms like ‘has’, ‘can’, and ‘should’ as well, but make sure not to give nouns just like you give for other variables, but treat boolean variables differently.
3. How to Name Private Variables or Functions/Methods
If you see an underscore (_) in front of a name, it implies that the variable, function or method is private.
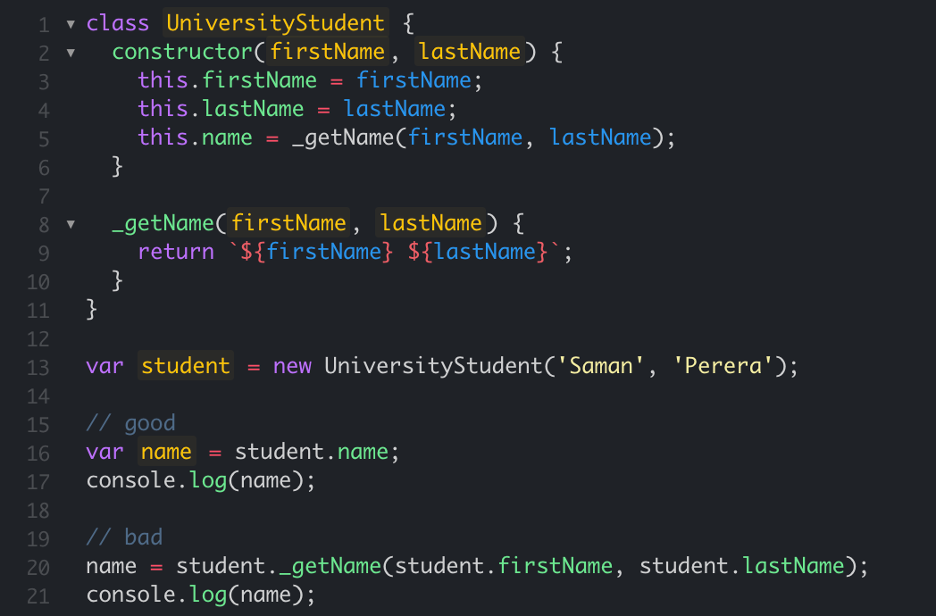
4. How to Name Constants?
Constants are the variables you declare with a value that is expected to remain unchanged. These variables are named with CAPITAL letters.

Before moving further, Let me refresh your knowledge…
Are you familiar with camelCase and PascalCase?
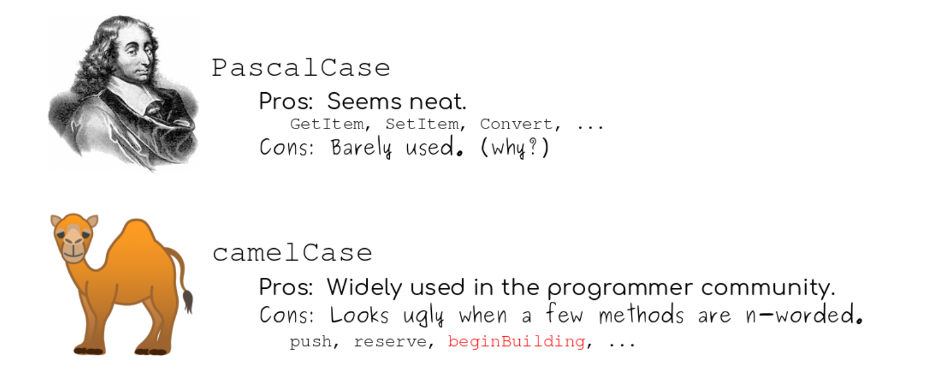
Clean Coding in JavaScript
High Quality Tech Tutorials and Guides (No Ads, Free)
Click Here to Register for the Newsletter5. How to Name JavaScript Functions?
This applies to JS Frameworks like Angular, React etc. and Backed Frameworks like Java SpringBoot, .NET etc.
When you are naming a Function/Method, use camelCase.
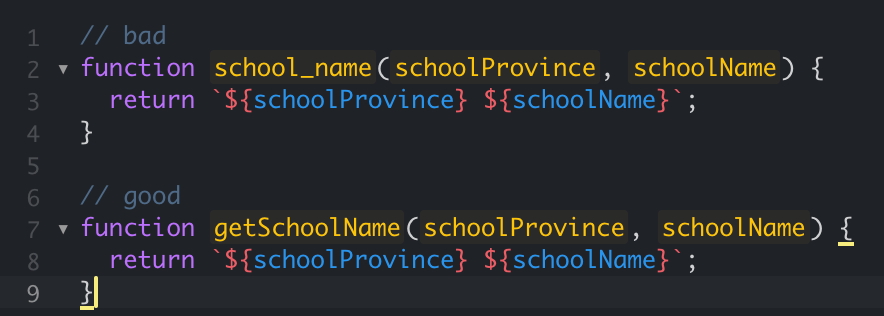
6. How to Name JavaScript Classes?
This applies to JS Frameworks like Angular, React etc. and Backed Frameworks like Java SpringBoot, .NET etc.
When you are naming a class, use PascalCase.
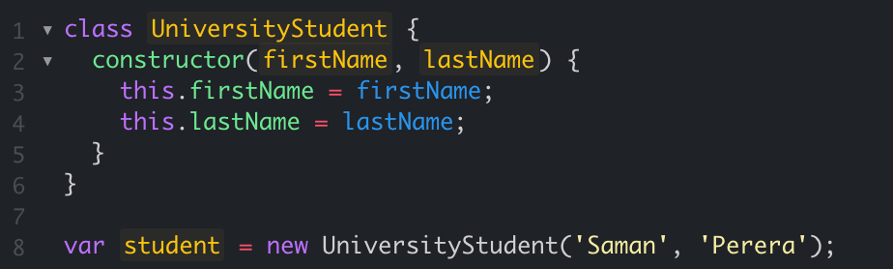
7. How to Name Components?
This applies to any JS Framework like Angular, React etc.
When you are naming a Component, use PascalCase. Components are common in modern JavaScript frameworks like Angular and React.
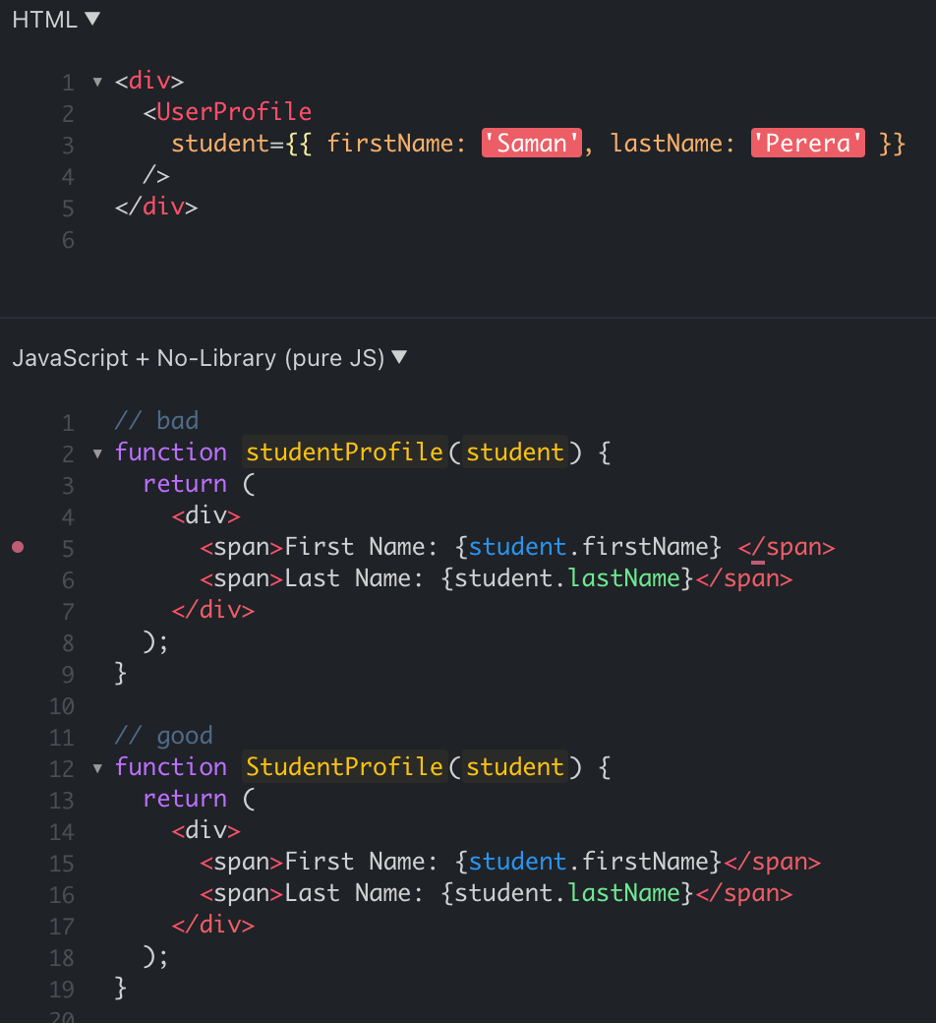
When you name your components in PascalCase, it is easier to identify them in HTML component bindings because HTML tags will be in simple letters, and your component will be in PascalCase.
8. Some General Tips
- Make sure to give pronounceable names for your identifiers.
- Avoid giving numbers in the middle of your code unexpectedly. Try to use variables appropriately.
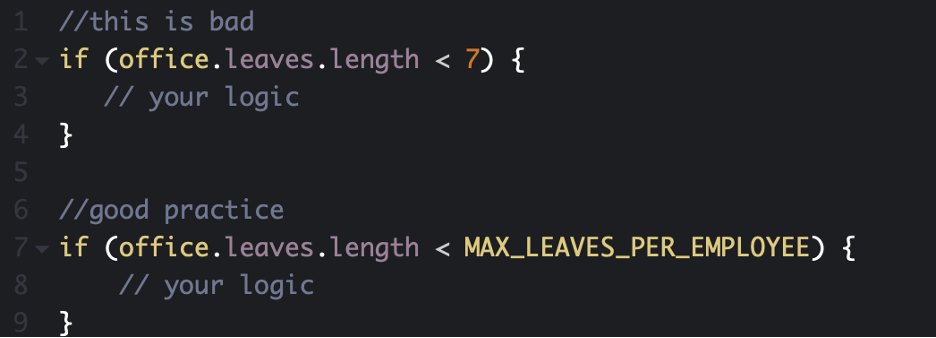
- When using axios or any other web service call and declaring your API path, don’t hardcode the path in every place where you call your backend APIs. Make sure to define your backend path as a base URL and import it whenever you need to call your API.
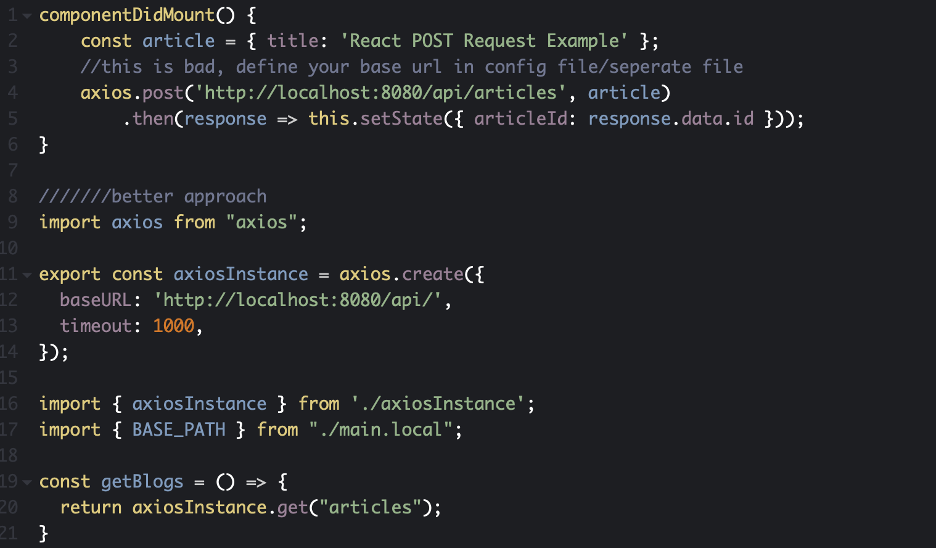
- Make your words/naming styles consistent across your application. For example, if you use getStudent(), getScores() kind of pattern for your methods/functions, don’t define some other similar functions like fetchTime(), fetchName() etc. Make them consistent as previous methods by naming getTime(), getName() etc.
- Make sure your functions/methods do just one thing. For example, calculateExamGrade() should do only calculate the Grade. But if it is calculating Grade AND saving the Grade in a database or sending an API request or even if it is performing a validation within the same calculateExamGrade method, it is ‘not nice’.
- Try to maintain a minimum number of arguments/parameters you pass to a function/method.
- Know your programming language’s style conventions. The best resource is to read the official style guides available. I’ll list some below for your reference.
Angular Style Guide: https://angular.io/guide/styleguide
React Style Guide: https://react-styleguidist.js.org
VueJS Style Guide: https://vuejs.org/v2/style-guide
Google Style Guide for Backend and Frontend Frameworks: https://google.github.io/styleguide
Summary
Clean Coding is a skill every developer should practice more often. This article presented some basic steps you can think of when you are coding next time. Clean Coding is not just about naming conventions, but I believe this is a very good starting point for anyone who is new to this topic. In the next article, I will write further details and aspects of clean coding.
Do you know that you can automate most of the above practices using some plugins? You can configure your IDE to ensure Clean Coding standards and easily check your coding style's compliance with official style guides. Let me know if you are interested to learn how to do it, So I’ll write further covering those aspects as well.
Don’t forget to subscribe to this blog for more tech articles like this!